If you are using Reflection and you wish you get property value using Reflection (string in this example) using C#, you can easily do it using the GetValue() method of the PropertyInfo class as shown below.
public static object GetPropertyValue(object source, string propertyName) { PropertyInfo property= source.GetType().GetProperty(propertyName); return property.GetValue(source, null); }
How to get the property value using reflection in C#?
Here’s the full code snippet demonstrating the retreival of the property value using reflection at runtime using C#.
using System; using System.Linq; using System.Reflection; namespace ConsoleApp1 { public class Employee { public string Name { get; set; } } class Program { private static Random random = new Random(); static void Main(string[] args) { Employee emp = new Employee(); emp.Name = "Senthil"; string propValue = GetPropertyValue(emp, "Name").ToString(); Console.WriteLine(propValue); Console.ReadLine(); } public static object GetPropertyValue(object source, string propertyName) { PropertyInfo property= source.GetType().GetProperty(propertyName); return property.GetValue(source, null); } } }
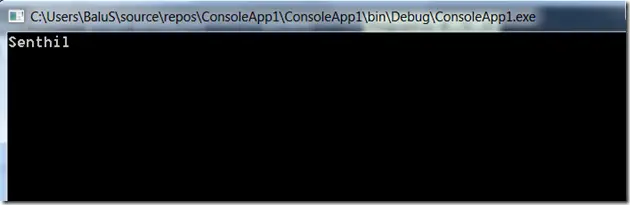