C# Compiler Error
CS0577 – The Conditional attribute is not valid on ‘function’ because it is a constructor, destructor, operator, or explicit interface implementation
Reason for the Error
You will get this error in your C# code when you have applied the conditional attributes to specific methods which are not supported by C#.
For example, let’s compile the below C# program
using System; namespace DeveloperPublishConsole1 { interface IEmployee { void GetData(); } public class MyClass : IEmployee { [System.Diagnostics.Conditional("a")] // CS0577 void IEmployee.GetData() { } } internal class Program { static void Main(string[] args) { Console.WriteLine("Hello World!"); } } }
You will receive the error code CS0577 because you have applied the conditional attribute on a method that is an explicit interface definition.
Error CS0577 The Conditional attribute is not valid on ‘MyClass.IEmployee.GetData()’ because it is a constructor, destructor, operator, lambda expression, or explicit interface implementation DeveloperPublishConsole1 C:\Users\SenthilBalu\source\repos\DeveloperPublishConsole1\DeveloperPublishConsole1\Program.cs 10 Active
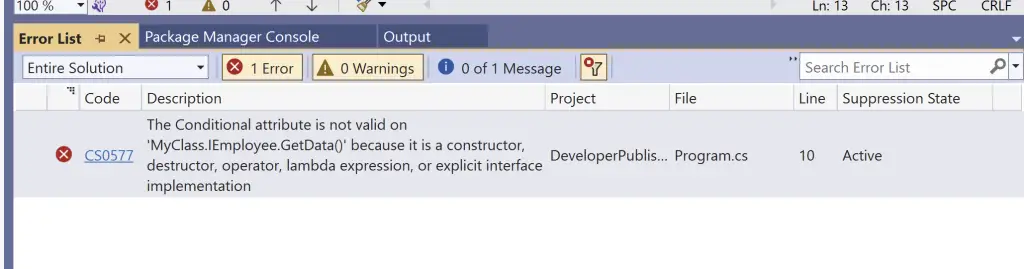
Solution
C# doesn’t allow you to have an conditional attributes applied on a explicit interface definition.
You can fix this error in your C# program by removing the conditional attribute on the GetData() method in the class MyClass.