C# Compiler Error
CS0542 – ‘user-defined type’ : member names cannot be the same as their enclosing type
Reason for the Error
There are multiple scenarios that will cause this error to appear in your C# program. These include
- The class contains the member with the same name as the class except the constructor.
- When you have a return type for a constructor.
Let’s look at our first example where the class contains the members with the same name as the class name.
using System; namespace DeveloperPublishConsoleCore { class Employee { // This returns the error code CS0542 public int Employee; } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
In the above program, Employee class contains a field named Employee. This will result in the C# error code CS0542.
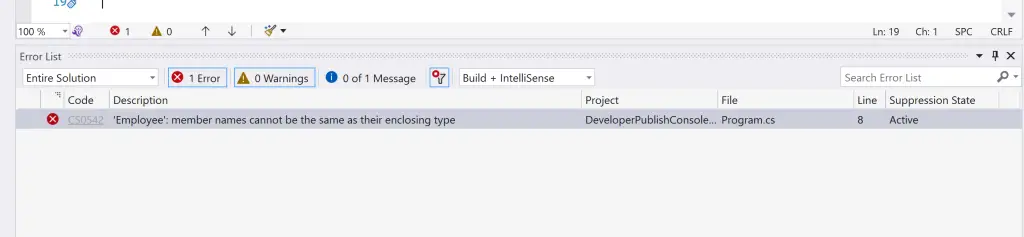
Here’s our second use case.
using System; namespace DeveloperPublishConsoleCore { class Employee { // This returns the error code CS0542 public int Employee() { return 0; } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
In the above program, the constructor in the Employee class returns a type which causes the error code CS0542.
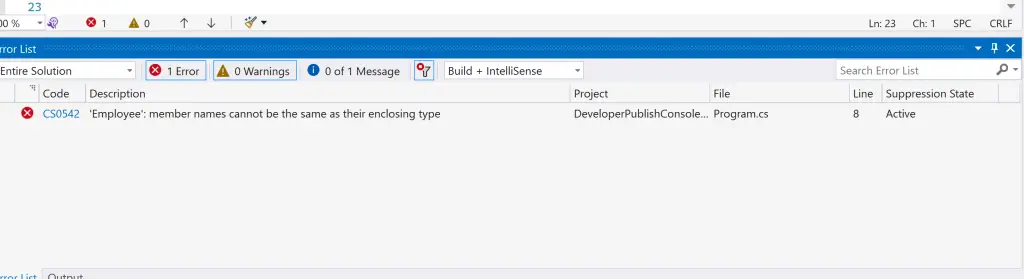
Solution
You can fix this error by ensuring that you either rename the field or the member that is causing the issue. For the constructors , ensure that you don’t have the return type.