C# Compiler Error
CS0529 – Inherited interface ‘interface1’ causes a cycle in the interface hierarchy of ‘interface2’
Reason for the Error
You’ll get this error in your C# code when you have an inheritance list of an interface with self references either directly or indirectly.
The inheritance list for an interface includes a direct or indirect reference to itself. An interface cannot inherit from itself.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishConsole1 { public interface Interface1 { } public interface Interface2 : Interface1, Interface3 { } public interface Interface3 : Interface2 { } internal class Program { static void Main(string[] args) { Console.WriteLine("No Error"); } } }
You’ll receive the error code CS0529 when run the above code snippet because you have included the interface Interface2 and Interface3 has a cyclic references in the inheritance list.
Error CS0529 Inherited interface ‘Interface3’ causes a cycle in the interface hierarchy of ‘Interface2’ DeveloperPublishConsole1 C:\Users\senth\source\repos\DeveloperPublishConsole1\DeveloperPublishConsole1\Program.cs 9 Active
Error CS0529 Inherited interface ‘Interface2’ causes a cycle in the interface hierarchy of ‘Interface3’ DeveloperPublishConsole1 C:\Users\senth\source\repos\DeveloperPublishConsole1\DeveloperPublishConsole1\Program.cs 13 Active
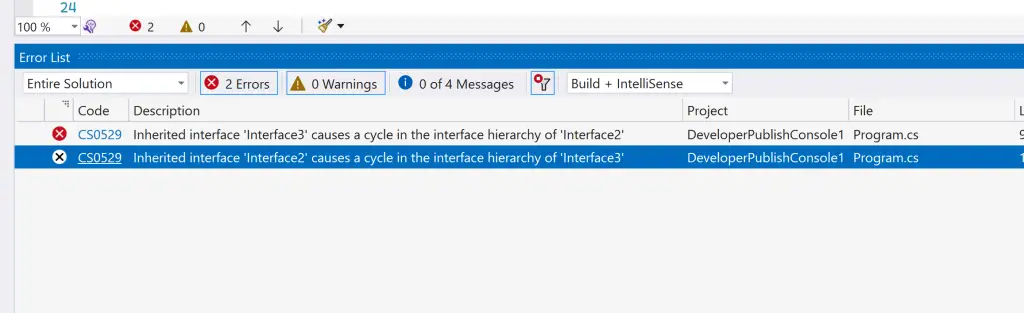
Solution
In C#, an interface cannot inherit from itself.
To fix this error in your C# code, you will need to remove the interface from the inheritance list that is causing the cyclic reference.