C# Compiler Error
CS0502 – ‘member’ cannot be both abstract and sealed
Reason for the Error
You’ll get this error in your C# code when you try to declare a class member as both abstract and sealed.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishNamespace { public abstract class Employee { public abstract void SetId(); } public class ContractEmployee : Employee { public abstract sealed override void SetId() { } } class Program { static void Main(string[] args) { Console.WriteLine("No Error"); } } }
You’ll receive the error code CS0502 because the C# compiler has detected that you have declared the SetId() in the ContractEmployee class as both abstract and sealed.
Error CS0502 ‘ContractEmployee.SetId()’ cannot be both abstract and sealed DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 10 Active
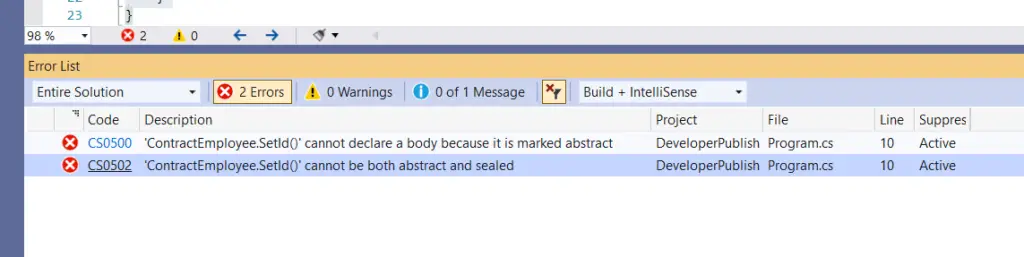
Solution
In C# programming language, you cannot declare a class member as both abstract and sealed. You can fix this error in your C# program by ensuring that the class member is either abstract or sealed but not both.