C# Compiler Error
CS0446 – Foreach cannot operate on a ‘Method or Delegate’. Did you intend to invoke the ‘Method or Delegate’?
Reason for the Error
You’ll get this error in your C# code when you are using the foreach statement and you use the method name without parentheses instead of a collection class.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishNamespace { class Program { static void Main(string[] args) { int[] array1 = new int[10]; // Results with the CS0446 foreach (int i in Test) { } Console.WriteLine("No Error"); } static void Test() { } } }
You’ll receive the error code CS0446 when you build the above C# code because you are using the method name “Test” without parentheses instead of using the array “array1”.
Error CS0446 Foreach cannot operate on a ‘method group’. Did you intend to invoke the ‘method group’? DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 10 Active
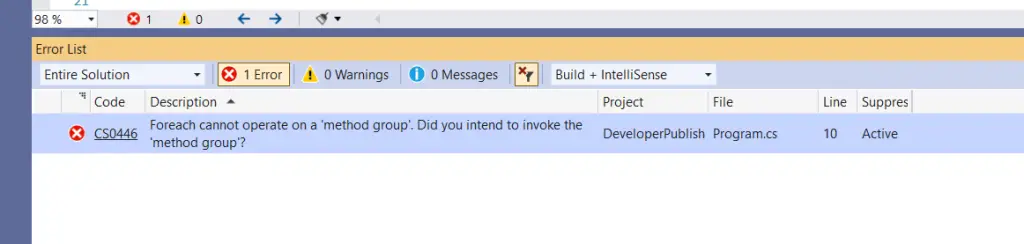
Solution
You can fix this error in your C# program by ensuring that you use a collection instead of a method with out parentheses. If you intend to use a function, ensure that the function returns a collection instead of void.
using System; namespace DeveloperPublishNamespace { class Program { static void Main(string[] args) { int[] array1 = new int[10]; // No Error now foreach (int i in Test()) { } Console.WriteLine("No Error"); } static int[] Test() { return new int[10]; } } }