C# Compiler Error
CS0269 – Use of unassigned out parameter ‘parameter’
Reason for the Error
You’ll get this error in your C# code when you are using the out parameter for a function and using it before assigning any value to it.
For example, lets try to compile the below code snippet.
using System; namespace DeveloperPubNamespace { class Program { public static void SetData(out int id) { int output = id; Console.WriteLine("output"); } public static void Main() { Console.WriteLine("No Error"); } } }
You’ll receive the error code CS0269 because you are using the out parameter “id” for the function SetData and accessing its value before assigning any value to it.
Error CS0269 Use of unassigned out parameter ‘id’ DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 9 Active
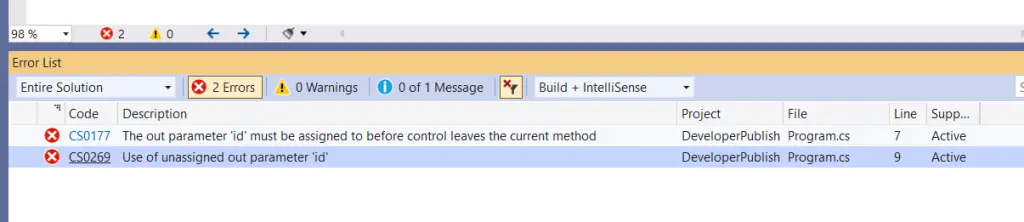
Solution
You can fix this error in your C# program in multiple ways. One possible solution is to use the ref keyword instead of the out. The other possible solution is to assign a value to the out parameter first before using them with-in your function.
using System; namespace DeveloperPubNamespace { class Program { public static void SetData(out int id) { id = 25; int output = id; Console.WriteLine("output"); } public static void Main() { Console.WriteLine("No Error"); } } }