C# Compiler Error
CS0220 – The operation overflows at compile time in checked mode
Reason for the Error
You will receive this error when the C# compiler detects that an operation will result in the data loss when the overflow checking (checked) is performed by the compiler.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Program { static void Main() { const int var1 = 1010004; const int var2 = 1010007; int var3 = (var1 * var2); // CS0220 } } }
This program will result with the error code CS0220 because C# compiler has detected that the expression that multiplies var1 and var2 will result in overflow at compile time.
Error CS0220 The operation overflows at compile time in checked mode ConsoleApp3 C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 10 Active
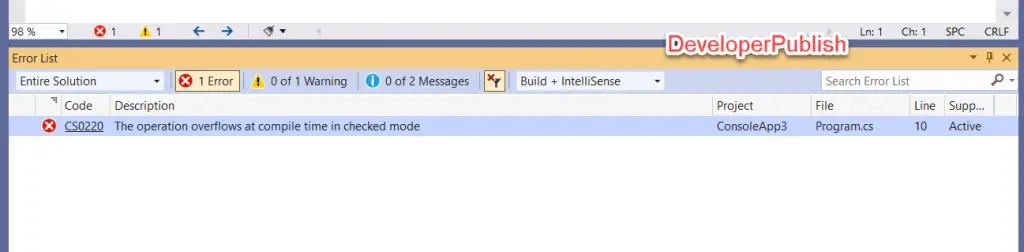
Solution
To fix the error code CS0220 in C#, you’ll either need to correct the inputs that are used for the operation or use the unchecked block to perform this perform that will return with data loss.
using System; namespace DeveloperPubNamespace { class Program { static void Main() { const int var1 = 1000000; const int var2 = 1000000; unchecked { int var4 = (var1 * var2); Console.WriteLine(var4); } } } }