C# Compiler Error
CS0205 – Cannot call an abstract base member: ‘method’
Reason for the Error
You will receive this error in your C# program when you are trying to call an abstract method.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { abstract public class BaseEmployee { abstract public void SetId(int i); } public class Employee : BaseEmployee { public override void SetId(int i) { base.SetId(i); } } class Program { static void Main() { } } }
This program will result with the error code CS0205 because the method SetId() in BaseEmployee is abstract and we are attempting to call this function via base.SetId() from Employee class.
Error CS0205 Cannot call an abstract base member: ‘BaseEmployee.SetId(int)’ ConsoleApp3 C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 12 Active
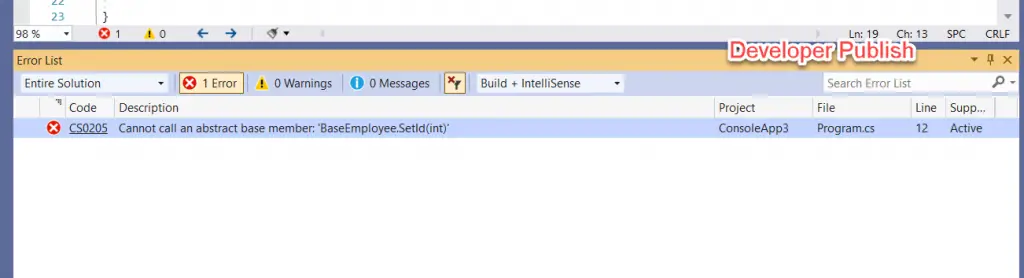
Solution
To fix the error code CS0205, you should avoid calling the abstract method as they don’t have a method body.