C# Compiler Error
CS0199 – Fields of static readonly field ‘name’ cannot be passed ref or out (except in a static constructor)
Reason for the Error
You will receive this error when you are trying to pass the static readonly field to a function outside of the constructor in your C# program.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Employee { public static readonly int Id; static void SetId(ref int refId) { refId = 0; } public void InitializeEmployee() { SetId(ref Id); // CS0199 } } class Program { static void Main(string[] args) { } } }
This program has a static readonly field Id in the Employee class. We are trying to pass this field to the SetId’s ref parameter inside the InitializeEmployee function. This results in the CS0199.
Error CS0199 A static readonly field cannot be used as a ref or out value (except in a static constructor) ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 12 Active
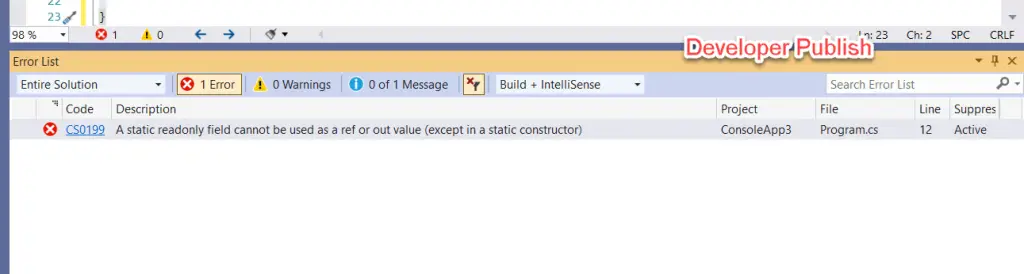
Solution
To fix the error code CS0199, You can will need to ensure that you call the ref or out parameter of the function by passing the readonly static field only with-in the static constructor. The other option is to revisit your readonly static field and making them static instead of readonly static.
namespace DeveloperPubNamespace { class Employee { public static readonly int Id; static void SetId(ref int refId) { refId = 0; } static Employee() { SetId(ref Id); // CS0192 } } class Program { static void Main(string[] args) { } } }