C# Compiler Error
CS0192 – Fields of static readonly field ‘name’ cannot be passed ref or out (except in a static constructor)
Reason for the Error
You will receive this error when you have declared a field as readonly and you try to pass the readonly field to a ref or out parameter outside of constructor in your C# code.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Employee { public readonly int Id; static void SetId(ref int refId) { refId = 0; } public void InitializeEmployee() { SetId(ref Id); // CS0192 } } class Program { static void Main(string[] args) { } } }
The above code snippet will result with the C# error code CS0192 because Id is a readonly field is been passed to the ref parameter of the SetId function.
Error CS0192 A readonly field cannot be used as a ref or out value (except in a constructor) ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 12 Active
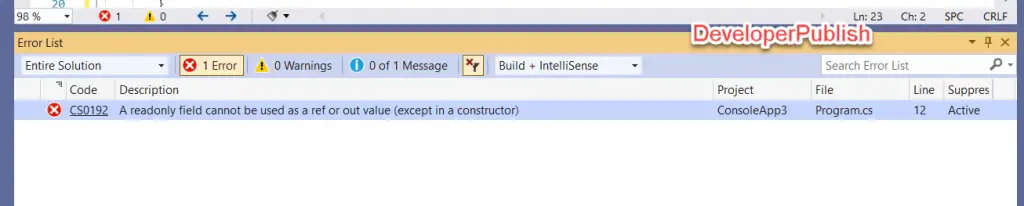
Solution
To fix the error, ensure that the readonly fields are not passed to the ref or out parameter to functions in your C# code. You can however pass the readonly field to out or ref parameter inside constructor.
Below is a sample code snippet demonstrating how to fix the error code CS0191.
namespace DeveloperPubNamespace { class Employee { public readonly int Id; static void SetId(ref int refId) { refId = 0; } public Employee() { SetId(ref Id); } } class Program { static void Main(string[] args) { } } }