C# Compiler Error
CS0191 – Property or indexer ‘name’ cannot be assigned to — it is read only
Reason for the Error
You will receive this error when you try to assign a value to the readonly property in a function. In C#, the readonly fields can only be assigned during the declaration or in a constructor.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Employee { public readonly int Id; Employee() { } public void InitializeEmployee(int id) { Id = id; } } class Program { static void Main(string[] args) { } } }
The above code snippet will result with the C# error code CS0191 because Id is a readonly field and this field is been assigned a value in the function InitializeEmployee.
Error CS0191 A readonly field cannot be assigned to (except in a constructor or init-only setter of the type in which the field is defined or a variable initializer) ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 13 Active
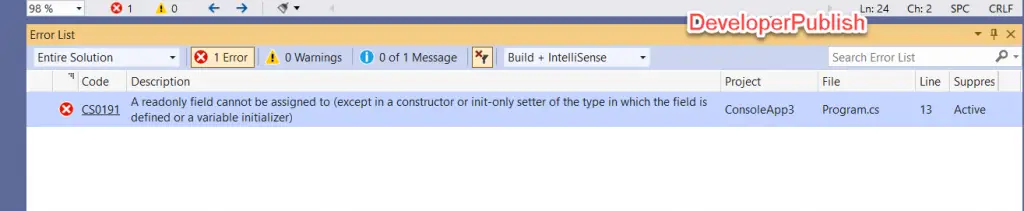
Solution
To fix the error, ensure that the readonly fields are only assigned inside the constructor or during the declaration in C#. Below is a sample code snippet demonstrating how to fix the error code CS0191.
namespace DeveloperPubNamespace { class Employee { public readonly int Id; Employee(int id) { Id = id; } public void InitializeEmployee(int id) { } } class Program { static void Main(string[] args) { } } }