C# Compiler Error
CS0181 – Attribute constructor parameter ‘value’ has type ‘decimal’, which is not a valid attribute parameter type
Reason for the Error
You will receive this error when you have used a unsupported type as parameter for attribute in C#. In C#, when you use a non-primitive type (eg : Decimal) with a attributes, you will see this error. More details about the attributes specification in C# can be found at https://github.com/dotnet/csharplang/blob/main/spec/attributes.md#attribute-specification
For example, lets try to compile the below code snippet.
namespace DeveloperPubNamespace { using System; class DecimalAttribute : Attribute { public DecimalAttribute(decimal value) { } } class Program { [Decimal(123m)] public int Property1 { get; set; } static void Main(string[] args) { } } }
The above code snippet will result with the error code CS0181 because the Property1 uses a attribute named Decimal which passes a non-primitive type(decimal).
Error CS0181 Attribute constructor parameter ‘value’ has type ‘decimal’, which is not a valid attribute parameter type ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 12 Active
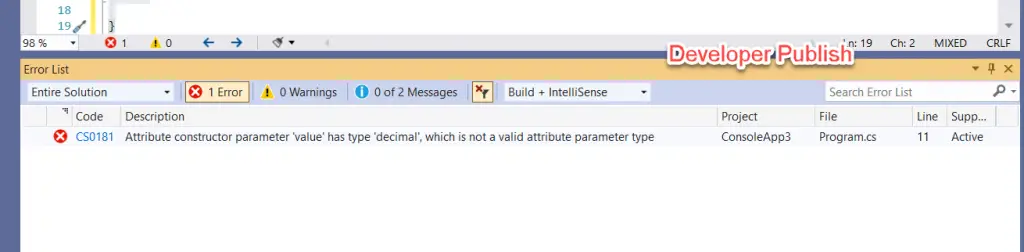
Solution
To fix the error, ensure that attributes uses the parameter types as defined in the specification. In the above example, try changing the parameter decimal to double for the DecimalAttribute constructor and pass the double value instead of decimal.
namespace DeveloperPubNamespace { using System; class DecimalAttribute : Attribute { public DecimalAttribute(double value) { } } class Program { [Decimal(123d)] public int Property1 { get; set; } static void Main(string[] args) { } } }