C# Compiler Error
CS0180 – ‘member’ cannot be both extern and abstract.
Reason for the Error
You will receive this error when you have used both abstract and extern keywords on a member. Both of these keywords (abstract and extern) are mutually exclusive in C#.
For example, lets try to compile the below code snippet.
namespace DeveloperPubNamespace { public class Class1 { // This will return with CS0180 Error. public extern abstract int Method1(int a); } class Program { static void Main(string[] args) { } } }
The above code snippet will result with the error code CS0180 because the Method1 is marked as both extern and abstract.
Error CS0180 ‘Class1.Method1(int)’ cannot be both extern and abstract ConsoleApp3 C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 7 Active
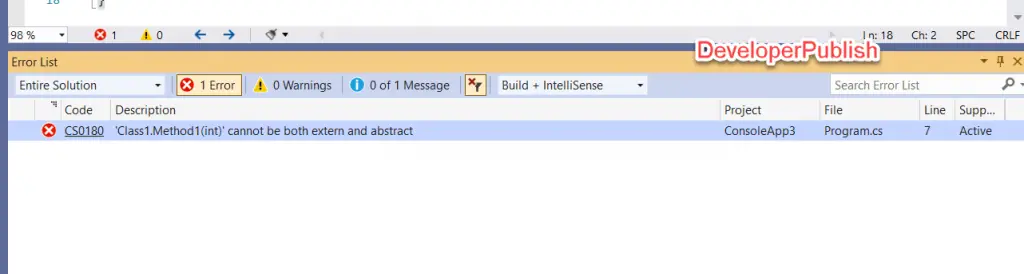
Solution
To fix the error, remove either the extern keyword abstract and change your logic accordingly. Below is a code snippet showing how the error is fixed by removing the extern.
namespace DeveloperPubNamespace { public class Class1 { public extern int Method1(int a); } class Program { static void Main(string[] args) { } } }