C# Compiler Error
CS0103 – The name ‘identifier’ does not exist in the current context
Reason for the Error
You will usually receive this error when you are trying to use a identifier name in C# that doesnot exist with-in the current scope. One of the simple example of this error is when you declare a variable with-in a try block and try to access it in the catch block.
In the below example, the variable i is declared inside the try block, so its scope is restricted to the try block. When you try accessing it in the catch block is when you will receive the error.
using System; public class DeveloperPublish { public static void Main() { try { int i = 1; int j = 0; int result = i / j; } catch(DivideByZeroException) { string message = i + " is divivided by zero"; Console.WriteLine(message); } } }
Error CS0103 The name ‘i’ does not exist in the current context ConsoleApp1 C:\Users\Senthil\source\repos\ConsoleApp1\ConsoleApp1\Program.cs 16 Active
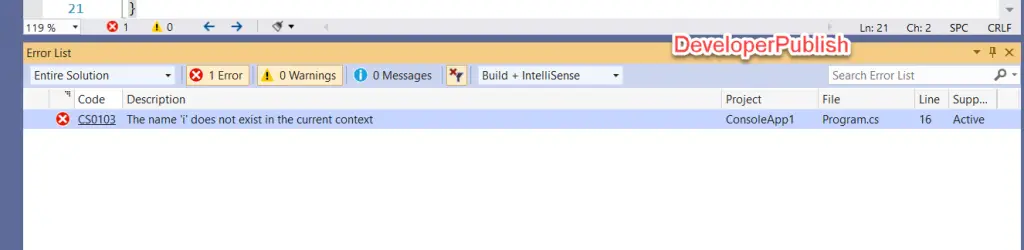
Solution
To fix the error move the declaration of the identifier that is causing the error to a higher scope so that it is available when it is accessed.
For example, to fix the error in the above code snippet, we just need to move the declaration of the identifier outside of the try block.
using System; public class DeveloperPublish { public static void Main() { int i = 1; try { int j = 0; int result = i / j; } catch(DivideByZeroException) { string message = i + " is divivided by zero"; Console.WriteLine(message); } } }