C# Compiler Error
CS0070 – The event ‘event’ can only appear on the left hand side of += or -= (except when used from within the type ‘type’)
Reason for the Error
You will receive this error when you have an event and try assigning it without an add or subtract references outside of where the event class is defined.
For example, try compiling the below code snippet.
public delegate void EventHandler(); public class classA { public event EventHandler Click; public static void OnClick() { EventHandler eventHandler; classA a = new classA(); eventHandler = a.Click; } } public class DeveloperPublish { public int Foo() { EventHandler eh = new EventHandler(classA.OnClick); classA a = new classA(); eh = a.Click; return 1; } public static void Main() { } }
This will result with the compiler error
Error CS0070 The event ‘classA.Click’ can only appear on the left hand side of += or -= (except when used from within the type ‘classA’)
Solution
Change the code eh = a.Click to include the += or -= as shown below to fix this error.
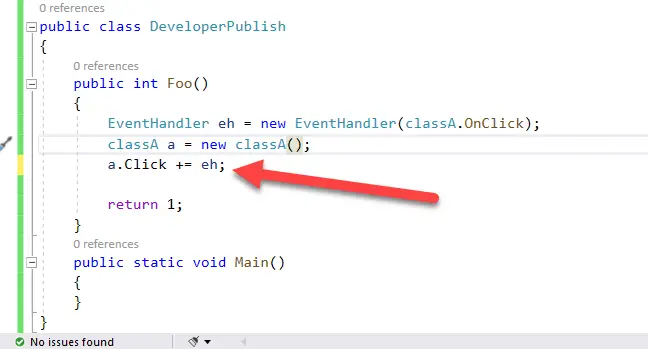