C# Compiler Error Message
CS0026 – Keyword ‘this’ is not valid in a static property, static method, or static field initializer
Reason for the Error
As the error message explains, this keyword refers to an object or the current instance. Since static methods are not associated to any instances of the class, you cannot use this keyword.
For example, try compiling the below code snippet.
using System; public class DeveloperPublish { public static int Id { get; set; } public static void Main() { this.Id = 1; Console.WriteLine(Id); } }
This will result in two errors.
Error CS0026 Keyword ‘this’ is not valid in a static property, static method, or static field initializer
Error CS0176 Member ‘DeveloperPublish.Id’ cannot be accessed with an instance reference; qualify it with a type name instead ConsoleApp1
The error CS0026 is the primary one we are targeting in this post.
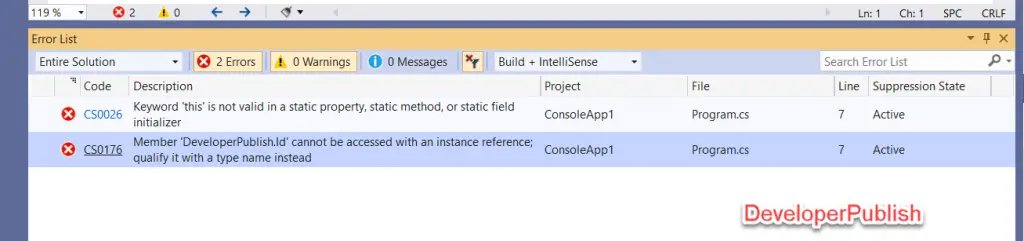
Solution
To fix this error, remove the this operator when accessing from the static class or access the static property as shown below.
using System; public class DeveloperPublish { public static int Id { get; set; } public static void Main() { DeveloperPublish.Id = 1; Console.WriteLine(Id); } }