This simple tip in C# shows you how to convert int to string by padding it with zeros. Assume that you have an integer value in C# that you will need to convert to string but with zeros prefixed with.
For example , You have an integer value containing 23 and you would like to display it as 0023.
How to Convert int to string with padding zeros in C#?
There are couple of options to get it work
- Use ToString with the Format Specifier D4
- Use String Interpolation in C# 6 and higher version.
Use ToString with the Format Specifier D4 in C#
Here’s a code snippet demonstrating how to use Format Specifier D4
public class Hello { public static void Main() { int input= 23; string result = input.ToString("D4"); System.Console.WriteLine(result); } }
Output
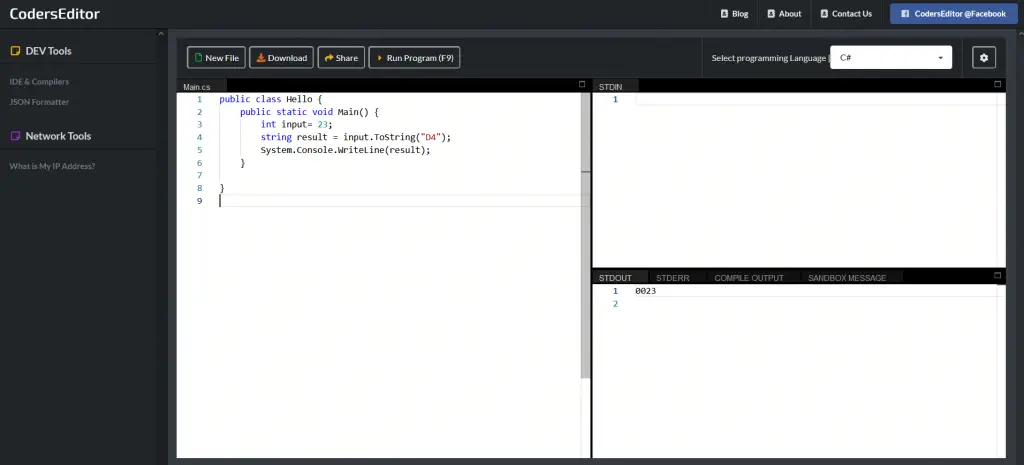
Use String Interpolation in C#
Here’s a code snippet demonstrating how to use string interpolation in C#.
public class Hello { public static void Main() { int input= 23; string result = $"{input:0000}"; System.Console.WriteLine(result); } }
Output
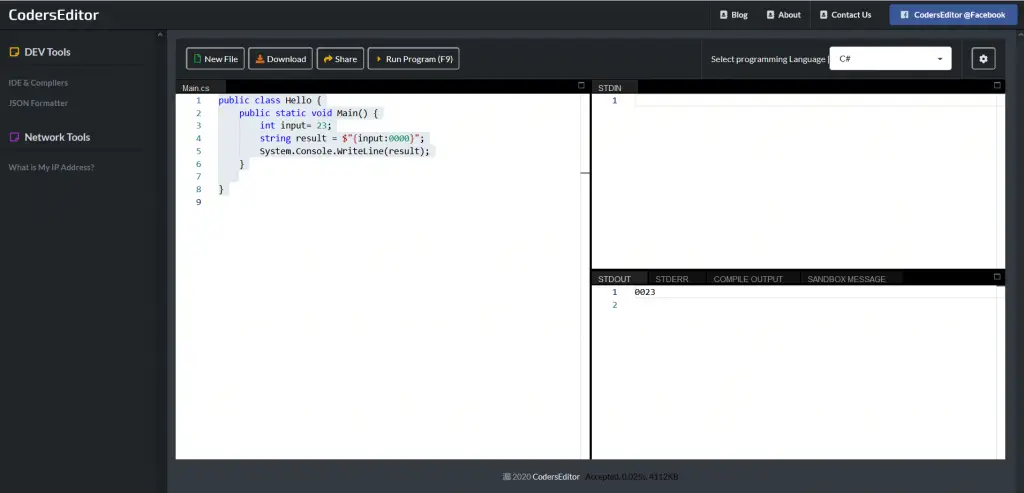