C# Compiler Error
CS0547 – ‘property’ : property or indexer cannot have void type
Reason for the Error
You will get this error in your C# code when you have a property or indexer with a return type of void.
For example, let’s compile the below C# program
using System; namespace DeveloperPublishConsoleCore { public class Employee { public void Id { get { // return 0; } } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }
You will receive the error code CS0547 because the class Employee contains a property Id that has a return type void.
Error CS0547 ‘Employee.Id’: property or indexer cannot have void type DeveloperPublishConsoleCore C:\Users\senth\source\repos\DeveloperPublishConsoleCore\DeveloperPublishConsoleCore\Program.cs 7 Active
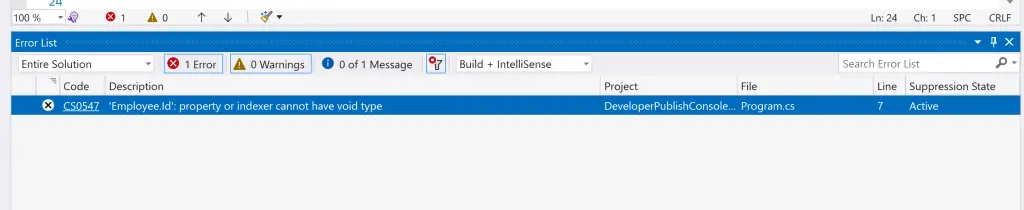
Solution
In C#, void is not a valid return type for a property or indexer. You can fix this error in your C# program by ensuring that a valid return type is provided for the property.
using System; namespace DeveloperPublishConsoleCore { public class Employee { public int Id { get { return 0; } } } internal class Program { static void Main(string[] args) { Console.WriteLine("DeveloperPublish Hello World!"); } } }