C# Compiler Error
CS0442 – ‘Property’: abstract properties cannot have private accessors
Reason for the Error
You’ll get this error in your C# code when you try to use the private access modifier on an accessor of a abstract property.
For example, let’s try to compile the below C# code snippet.
using System; namespace DeveloperPublishNamespace { public abstract class Employee { public abstract int Id { get; private set; } } class Program { static void Main(string[] args) { Console.WriteLine("Hello World!"); } } }
You’ll receive the error code CS0442 when you build the above C# code because you have declared an abstract property “Id” in the Employee class but you have modified the access modifier of the setter to private.
Error CS0442 ‘Employee.Id.set’: abstract properties cannot have private accessors DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 9 Active
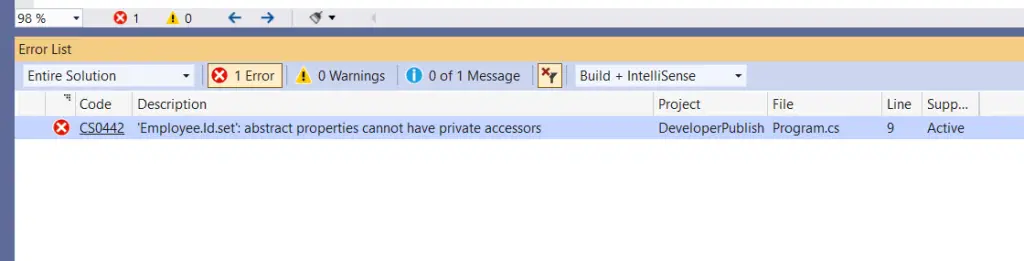
Solution
You can fix this error in your C# program by either making the accessor non-abstract or using a different access modifier for the property.
using System; namespace DeveloperPublishNamespace { public abstract class Employee { public abstract int Id { get; set; } } class Program { static void Main(string[] args) { Console.WriteLine("Hello World!"); } } }