C# Compiler Error
CS0432 – Alias ‘identifier’ not found
Reason for the Error
You’ll get this error in your C# code when you attempt to use the “::” operator to the right hand side of the identifier that is not an alias.
For example, try compiling the below C# code snippet.
using System; namespace DeveloperPublishNamespace { public class Class1 { public class Class2 { public static void Function1() { Console.WriteLine("Inside Function1"); } } } class Program { static void Main(string[] args) { A::Class2.Function1(); Console.WriteLine("Hello World!"); } } }
You’ll receive the error code CS0432 when you try to compile the above C# program because you are using the :: operator to the right of the identifier “A” where you don’t have the alias “A” defined.
Error CS0432 Alias ‘A’ not found DeveloperPublish C:\Users\Balu\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 20 Active
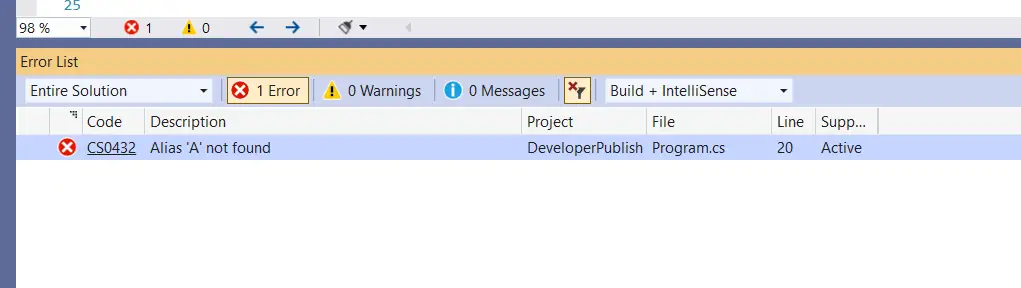
Solution
You can fix this error in your C# program by using the “.” operator instead of “::” operator and define the alias as shown below.
using System; namespace DeveloperPublishNamespace { using A = Class1; public class Class1 { public class Class2 { public static void Function1() { Console.WriteLine("Inside Function1"); } } } class Program { static void Main(string[] args) { A.Class2.Function1(); Console.WriteLine("Hello World!"); } } }