C# Compiler Error
CS0263 – Partial declarations of ‘type’ must not specify different base classes
Reason for the Error
You’ll get this error in your C# code when you have defined the partial type with same name and each one of them has a different base class.
For example, lets try to compile the below code snippet.
using System; namespace DeveloperPubNamespace { public class BaseClass1 { public string Name { get; set; } } public class BaseClass2 { public string Name { get; set; } } public partial class class1 : BaseClass2 { } public partial class class1 : BaseClass1 { } class Program { public static void Main() { Console.WriteLine("Welcome"); } } }
You’ll receive the error code CS0263 when you try to build the above C# program because you have declared multiple partial types “class1” but each one inheriting from a different base class “BaseClass1” and “BaseClass2”
Error CS0263 Partial declarations of ‘class1’ must not specify different base classes DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 15 Active
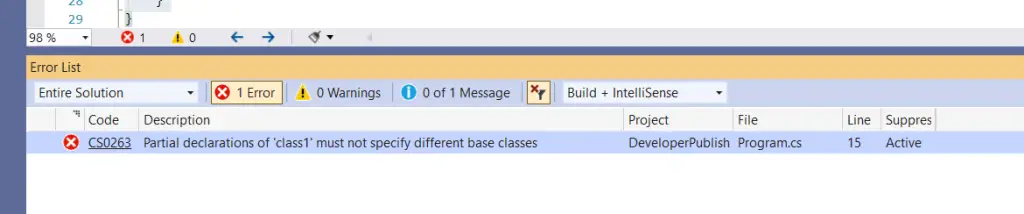
Solution
When you define a type in partial declarations in your C# program, it is important to specify the same base types for each of the partial types.
You can fix the above program by specifying the same base class for both the partial type declarations as shown below.
using System; namespace DeveloperPubNamespace { public class BaseClass1 { public string Name { get; set; } } public class BaseClass2 { public string Name { get; set; } } public partial class class1 : BaseClass1 { } public partial class class1 : BaseClass1 { } class Program { public static void Main() { Console.WriteLine("Welcome"); } } }