C# Compiler Error
CS0236 – A field initializer cannot reference the non-static field, method, or property ‘name’
Reason for the Error
You will receive this error in your C# program when you are trying to initialize a member or variable using other instance fields outside of method.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { public class Employee { public string SurName = ""; public string LastName = SurName; } class Program { public static void Main() { } } }
This program will result with the C# error code CS0236 because the instance field LastName is initialized directly (outside of method) using another instance field SurName.
Error CS0236 A field initializer cannot reference the non-static field, method, or property ‘Employee.SurName’ DeveloperPublish C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 6 Active
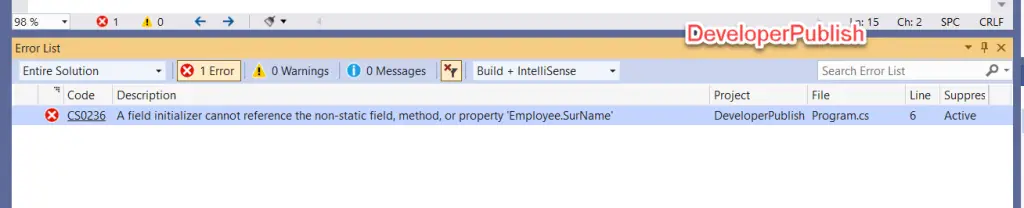
Solution
To fix the error code CS0236 in C#, you should consider initializing the field either inside a method or using the class’s constructor.
namespace DeveloperPubNamespace { public class Employee { public string SurName = ""; public string LastName; public Employee() { LastName = SurName; } } class Program { public static void Main() { } } }