C# Compiler Error
CS0221 – Constant value ‘value’ cannot be converted to a ‘type’ (use ‘unchecked’ syntax to override)
Reason for the Error
You will receive this error when the C# compiler detects that an assignment operation will result with data loss. This happens because by default checked block is used in .NET.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Program { static void Main() { int input = (int)9999999999; } } }
This program will result with the error code CS0221 because C# compiler has detected that there is an assignment of a constant value that will result with the overflow value.
Error CS0221 Constant value ‘9999999999’ cannot be converted to a ‘int’ (use ‘unchecked’ syntax to override) ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 8 Active
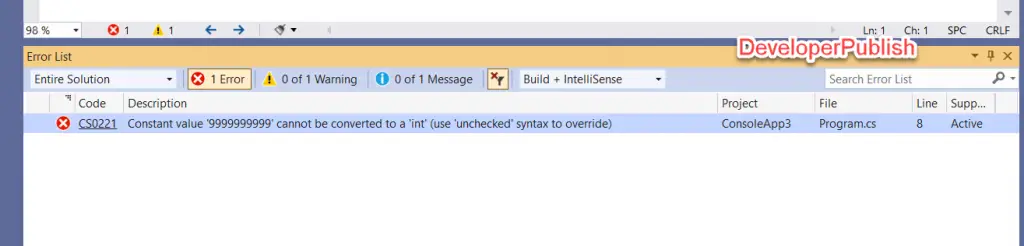
Solution
To fix the error code CS0220 in C#, you’ll either need to correct the value that is assigned or use the unchecked block to perform this operation.
namespace DeveloperPubNamespace { class Program { static void Main() { unchecked { int input = (int)9999999999; } } }