C# Compiler Error
CS0200 – Property or indexer ‘property’ cannot be assigned to — it is read only
Reason for the Error
You will receive this error in your C# code when you attempt to assign a value to a property that does not have a set accessor and the assignment of the value was made outside the constructor.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { class Employee { public int Id { get; } } class Program { static void Main(string[] args) { Employee emp = new Employee(); emp.Id = 1; } } }
This program has a class “Employee” with the Property “Id” that doesnot have a set accessor. We are attempting to assigning the value 1 to the Id via the instance inside the Main method.
This results with the error code CS0200.
Error CS0200 Property or indexer ‘Employee.Id’ cannot be assigned to — it is read only ConsoleApp3 C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 12 Active
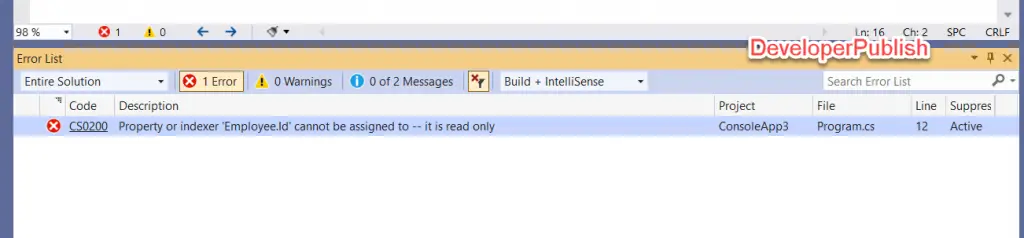
Solution
To fix the error code CS0200, you should either add the set accessor or else assign the value via the constructor.
namespace DeveloperPubNamespace { class Employee { public int Id { get; } public Employee() { Id = 1; } } class Program { static void Main(string[] args) { } } }