C# Compiler Error
CS0188 – The ‘this’ object cannot be used before all of its fields are assigned to
Reason for the Error
You will receive this error when you are using struct in your C# code and have not initialized the backing fields in the constructor. You might be initializing the property which would be causing this error. All the fields in a struct should be assigned by the constructor.
For example, try to compile the below code snippet.
using System; namespace DeveloperPubNamespace { public struct Employee { private int _id ; private string _name; public int Id { get { return this._id; } set { this._id = value; } } public Employee(int Id, string Name) { this.Id = Id; } } class Program { static void Main(string[] args) { } } }
Error CS0188 The ‘this’ object cannot be used before all of its fields have been assigned ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 18 Active
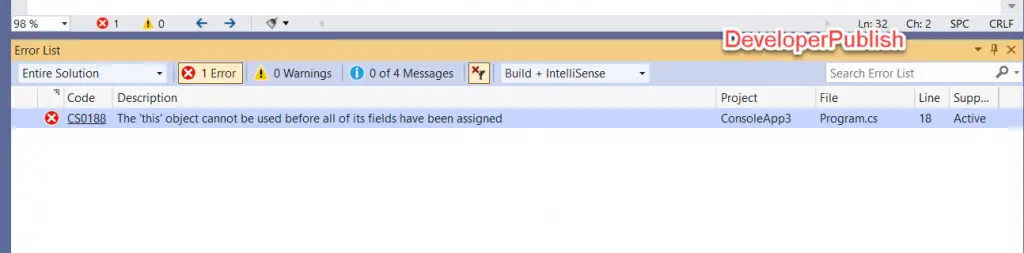
Solution
To fix the error, you will need to initialize all the backing fields of the property instead of initializing the property itself as shown below.
using System; namespace DeveloperPubNamespace { public struct Employee { private int _id ; private string _name; public int Id { get { return this._id; } set { this._id = value; } } public Employee(int Id, string Name) { _id = Id; _name = Name; } } class Program { static void Main(string[] args) { } } }