C# Compiler Error
CS0175 – Use of keyword ‘base’ is not valid in this context
Reason for the Error
You will receive this error when you have used the base keyword without specifying any member of the base class.
For example, try compiling the below code snippet
namespace ConsoleApp3 { using System; class BaseEmployee { public int Id = 0; } class Employee : BaseEmployee { public void DisplayEmployeeId() { // CS0175 Console.WriteLine(base); } } class Program { static void Main(string[] args) { Employee aClass = new Employee(); aClass.DisplayEmployeeId(); } } }
The above code snippet will result with the error code CS0175
Error CS0175 Use of keyword ‘base’ is not valid in this context ConsoleApp3 C:\Users\Senthil\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 13 Active
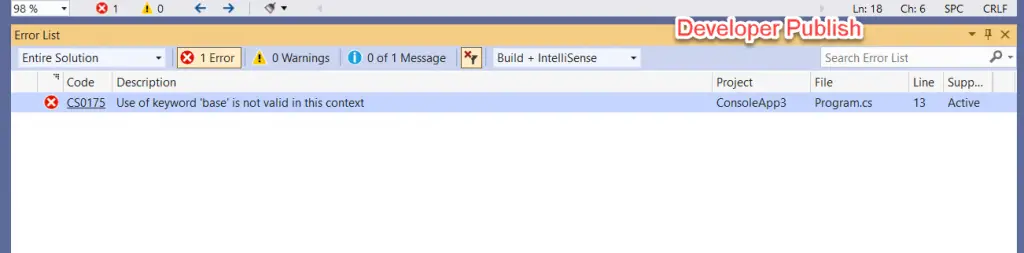
Solution
To fix this error, ensure that you specify the member of the base class when you use the base keyword. For example, the above code can be fixed by replacing the DisplayEmployeeId function with the below code snippet.
public void DisplayEmployeeId() { Console.WriteLine(base.Id); }